How to Inject service in AutoMapper profile class
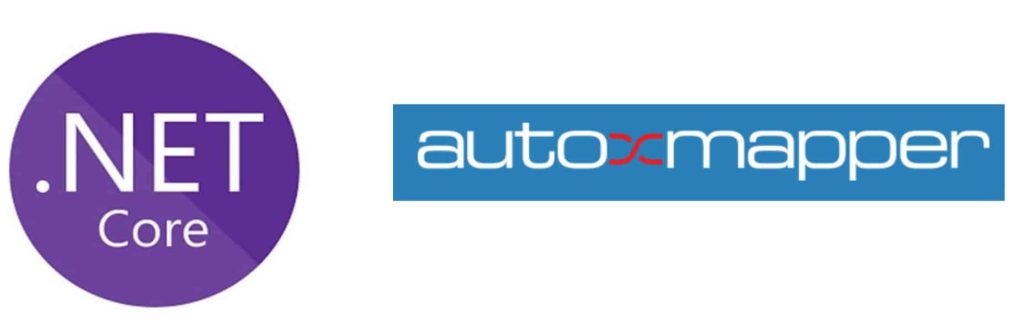
Today in this article, we will see how to Inject service in AutoMapper profile class. While setting up the Automapper profile during Startup services bootstrap you might want to use services within in Automapper profile classes.
Today in this article, we will cover below aspects,
It’s easy to configure services using the explicit dependency injection principle.
Service injection into the AutoMapper profile can be done and it’s done very rarely. But if you have valid use cases and want to perform some conditional mapping or want to consumer values of injected services from other modules or middleware then the below approach is very simple.
As we already discussed in our last article,
AutoMapper is an object mapper that helps you transform one object of one type into an output object of a different type. This type of transformation is often needed when you work on business logic.
Automapper helps to centralize your repeated boring mapping logic, address the separation of concerns, provide better control on mapping making your code clean and easy to maintain.
Here the idea with Automapper usage is simple, You have source type and destination type. You just transform object values from one source to the destination object. All you need is two types and profile registration. We will see what is profile registration in detail below.
For more details on dependency Injection of the IMapper interface is explained here already with more details,
Define the Automapper Profile with service Dependency Injection
Automapper Profile with service Dependency Injection can be defined as below.
Below example “SourceMappingProfile” is a profile class with constructor injection of service instance type i.e IEmployeeType.
Below I am defining the conditional profile for Automapper depending on the IEmployeeType.
Example
public class SourceMappingProfile : Profile
{
private IEmployeeType _employeeType;
public SourceMappingProfile()
{
}
public SourceMappingProfile(IEmployeeType employeeType)
{
_employeeType = employeeType;
if (_employeeType.empType == EmpType.Contract)
{
CreateMap<Source, Destination>()
.ForMember(dest => dest.Name, o => o.MapFrom(src => src.FirstName))
.ForMember(dest => dest.Location, o => o.MapFrom(src => src.Address))
.ForMember(dest => dest.Employee, o => o.MapFrom(src => src.Employee))
.ForMember(dest => dest.Identity, opt =>
{
opt.PreCondition(src => (src.Id != null));
opt.MapFrom(src => src.Id);
});
}
if (_employeeType.empType == EmpType.Permanent)
{
CreateMap<Source, Destination>()
.ForMember(dest => dest.Name, o => o.MapFrom(src => src.FirstName));
}
}
}
Define ConfigServices in Startup
I have defined the Define ConfigServices in Startup as below,
Example
services.AddScoped<ValidateInputAttribute<string>>();
services.AddScoped<IUniqueIdService, UniqueIdService>();
services.AddScoped<IEmployeeType,EmployeeType>();
services.AddSingleton(provider => new MapperConfiguration(cfg =>
{
cfg.AddProfile(new SourceMappingProfile(provider.CreateScope().ServiceProvider.GetService<IEmployeeType>()));
}).CreateMapper());
}
Above we are using scoped service instance. While scope service instances are created once per request it is often recommended they are resolved using a scoped instance of Application Builder. Then followed by using a service provider one can resolve the dependencies.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.